I Built A Useful PHP Package to Extract Styles in Custom WordPress Blocks
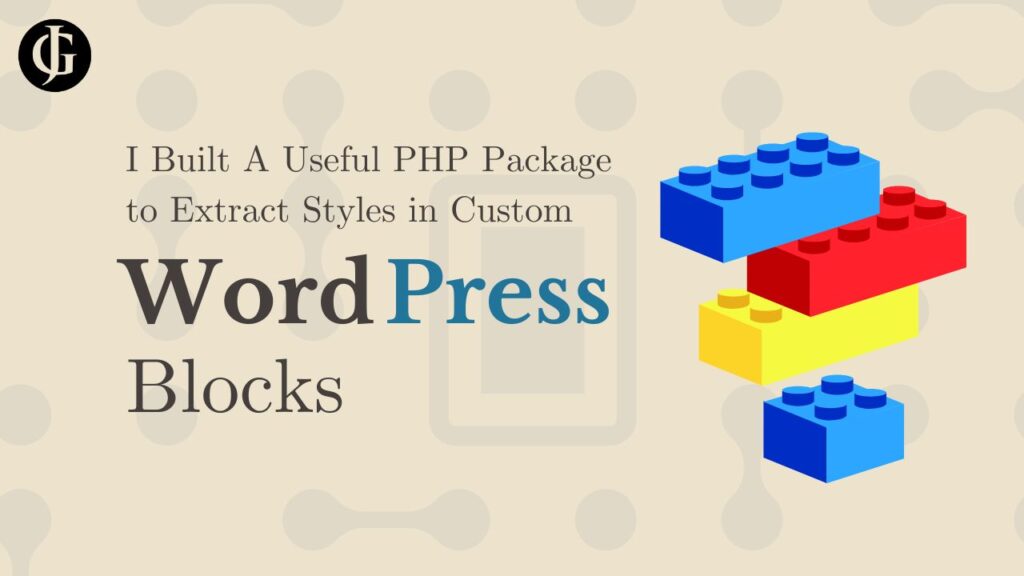
If you have ever developed custom blocks for WordPress, you have surely run into the problem of applying block styles to specific html elements of your block. Maybe you want to apply a text color to one p tag in your block, but not another. Or maybe, you want the border color block support to apply only to a wrapper around a text box and button, but not around the text box and button themselves (like in the core search block). The problem is, block supports are passed from the editor (edit.js) file to the render (render.php) file in an unintuitive way.
$attributes = [
'textColor' => '#123456',
'backgroundColor' => '#654321',
'fontSize' => '12px',
'style' => [
'color' => [
'text' => 'var(--wp--preset--color--text--primary)',
],
'elements' => [
'button' => [
'color' => [
'text' => '#987654',
'background' => '#123456'
]
]
]
]
];
This is an example of what the $attributes array that is passed from the edit script to the render script might look like. Obviously, there are dummy values here, but the structure is the key. So what’s so unintuitive about this? Well, depending on whether you choose a preset value or a fully custom, the entry for a particular block support might appear in a different place in the $attributes array.
For example, let’s say we want to get access to the value set for the block’s background color. If a custom color was selected, the value will be found at $attributes[‘style’][‘color’][‘background’] location in the $attributes array. But, if a WordPress preset was chosen, it will be found at the $attributes[‘backgroundColor’] location in the $attributes array. But, the kicker here is the only way to tell which is the case for this block is to check both locations. This is made more complex when you consider that other block supports, such as button color, text size, and padding operate similarly, but they hide their values at different locations within the array. In order to use these styles effectively, we need to know where to find all these style values, and create an intuitive interface for extracting them.
My Solution
My solution to this problem is JGWPBlockStyles. It is a php package that handles the extraction of block supports styles from the $attributes array in a smooth, intuitive interface. All you have to do is import the BlockStyle class, pass the $attributes array in, and then call the helper functions of the class that relate to each block supports style.
//import the class
use jtgraham38\jgwordpressstyle\BlockStyle;
//use a style parser to get the button styles
$style = new BlockStyle($attributes);
//get the font size block supports style value
$fontSize = $style->fontSize();
$fontSizeValue = $fontSize->value;
$fontSizeIsPreset = $fontSize->isPreset;
As you can see, it is quite simple. All you need to do is call the fontSize() method of the BlockStyle class, and it will return an object with two fields: value and isPreset. These fields are pretty self-explanatory: value gives the value of the block supports style… in this case, a font size. isPreset, meanwhile, is true if a WordPress preset value was chosen in the block editor, or false if a custom value was selected.
Under the Surface
I designed this whole package to be very easily extensible to invite feedback and contribution from the community. I did this because there are a lot of block supports, and right now, only a small subset of them are supported. But, thanks to the modular design of the package, support for a new block support can be easily added in its own file, and added to the BlockStyle class easily in a single line.
The BlockStyle class is a simple class that saves the $attributes array, and contains a few non-essential helper functions. So where is the style extraction defined? Well, the class implements a host of traits defined elsewhere in the package:
class BlockStyle{
use Typography;
use Color;
use ButtonColor;
...
}
Each of these traits contains the implementation for the extraction of a single block supports style or group of related styles. These traits go into the stored $attributes array, find where the value for the style is stored, and return the style value, along with whether it is a preset value or not.
The neat thing about structuring the package this way is that, to add a support for a new block supports style, all we need to do is define a new trait, and add a use TraitName; line to the BlockStyle class. Then, the class will be able to extract that style using the new implementation.
Contribution Welcome!
JGWPBlockStyles is a project I built because, after building a few custom block types for WordPress (see here or here), I quickly realized how big of a headache writing code to extract block supports style from the $attributes array to apply to specific html elements in the render file was. It was a lot of boilerplate, repeat code. This was my solution. But, it only has support for a small number of all the block supports that the WordPress block editor contains. I plan to add support for new ones as I need them, but, I would like to open this package up to contribution from others. Open source contribution will make developing custom block types easier for everyone, leading to a better block development experience, and a more expansive selection of blocks for WordPress users everywhere to use on their sites.
With that in mind, please visit the project github, fork, add a trait for a new block supports style, recommend structure change, or anything at all to make the project better. Thanks for reading and contributing! I hope the package improves your block development experience!
Leave a Reply